In this Azure AI Vision blog post, we will show how to extract text from images using Azure AI Vision and Azure SDK for .NET.
- Azure AI Vision allows us to analyse images and perform operations against objects inside images. AI Vision capabilities include:
- Optical Character Recognition (OCR) allows us to extract text from images, whether they are printed or handwritten, as we will see in this post.
- Extract visual features, generate captions, and identify faces and objects.
- Recognise human faces for facial recognition software, including image blurring and access control.
To perform AI operations on images, we use the official Microsoft Azure SDK for .NET. The AI Vision library is called Azure.AI.Vision.ImageAnalysis
.
Deploy Azure AI Vision Resource
Before accessing the AI Vision service, we need to deploy an Azure resource that will give us access to the service. To deploy the service, we can either use the Azure portal, Azure PowerShell, Azure CLI, or the .NET SDK for Azure. In our case, we will use Azure Bicep with the following configuration.
resource aivision 'Microsoft.CognitiveServices/accounts@2023-05-01' = {
name: 'CognitiveServices'
location: 'southeastasia'
sku: {
name: 'S0'
}
kind: 'ComputerVision'
properties: {
}
}
Once the service is deployed, Open the resource from the Azure portal and note the API Key and Endpoint.
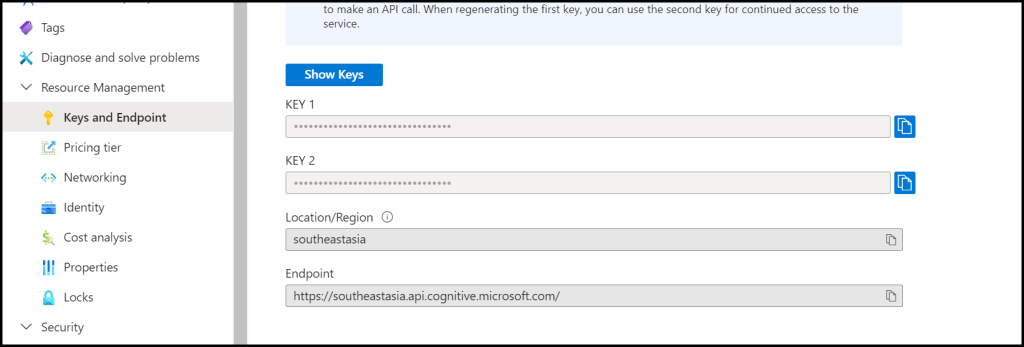
Program
Now that we have the service deployed to Azure and the access details to the service, we can use the AI Vision library to detect an image and give us a description of it. Start by creating a C# console application and installing the Vision library.
dotnet add package Azure.AI.Vision.ImageAnalysis --version 1.0.0-beta.3
In the program root directory, create an Images directory and copy all the images from which you need AI Vision to extract text.
using System;
using System.IO;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Text.Json;
using System.Threading.Tasks;
using System.Drawing;
using Microsoft.Extensions.Configuration;
using Azure;
using Azure.AI.Vision.ImageAnalysis; // AI Vision namespace
public class Program
{
static void Main()
{
AnalyzeImages();
}
static void AnalyzeImages()
{
// Get config settings from AppSettings
IConfigurationBuilder builder = new ConfigurationBuilder().AddJsonFile("appsettings.json");
IConfigurationRoot configuration = builder.Build();
string aiSvcEndpoint = configuration["AIServicesEndpoint"];
string aiSvcKey = configuration["AIServicesKey"];
ImageAnalysisClient client = new ImageAnalysisClient(
new Uri(aiSvcEndpoint),
new AzureKeyCredential(aiSvcKey));
string[] imageFiles = Directory.GetFiles("images");
foreach (string imageFile in imageFiles)
{
using FileStream stream = new FileStream(imageFile, FileMode.Open);
ImageAnalysisResult result = client.Analyze(
BinaryData.FromStream(stream),
VisualFeatures.Read);
foreach (DetectedTextBlock block in result.Read.Blocks)
foreach (DetectedTextLine line in block.Lines)
{
Console.WriteLine($" Line: '{line.Text}'");
foreach (DetectedTextWord word in line.Words)
{
Console.WriteLine($" Word: '{word.Text}', Confidence {word.Confidence.ToString("#.####")}");
}
}
}
}
}
Before you run the program, add your Azure AI Vision resource Key and Endpoint to an appsettings.json file.
In the example below, I will extract the text from the image above (from the Azure portal).
Line: 'to make an API call. When regenerating the first key, you can use the second key for continued access to the'
Word: 'to', Confidence .999
Word: 'make', Confidence .984
Word: 'an', Confidence .998
Word: 'API', Confidence .958
Word: 'call.', Confidence .996
Word: 'When', Confidence .993
Word: 'regenerating', Confidence .994
Word: 'the', Confidence .999
Word: 'first', Confidence .994
Word: 'key,', Confidence .987
Word: 'you', Confidence .999
Word: 'can', Confidence .998
Word: 'use', Confidence .993
Word: 'the', Confidence .999
Word: 'second', Confidence .996
Word: 'key', Confidence .998
Word: 'for', Confidence .998
Word: 'continued', Confidence .994
Word: 'access', Confidence .996
Word: 'to', Confidence .999
Word: 'the', Confidence .999
Line: 'Tags'
Word: 'Tags', Confidence .991
Line: 'service.'
Word: 'service.', Confidence .994
Line: 'X Diagnose and solve problems'
Word: 'X', Confidence .578
Word: 'Diagnose', Confidence .994
Word: 'and', Confidence .999
Word: 'solve', Confidence .998
Word: 'problems', Confidence .993
Line: 'Show Keys'
Word: 'Show', Confidence .993
Word: 'Keys', Confidence .99
Line: 'V Resource Management'
Word: 'V', Confidence .882
Word: 'Resource', Confidence .994
Word: 'Management', Confidence .993
Line: 'KEY 1'
Word: 'KEY', Confidence .998
Word: '1', Confidence .959
Line: 'Keys and Endpoint'
Word: 'Keys', Confidence .993
Word: 'and', Confidence .999
Word: 'Endpoint', Confidence .993
Line: '...'
Word: '...', Confidence .959
Line: '.......................'
Word: '.......................', Confidence .782
Line: 'Pricing tier'
Word: 'Pricing', Confidence .751
Word: 'tier', Confidence .993
Line: 'KEY 2'
Word: 'KEY', Confidence .998
Word: '2', Confidence .997
Line: '( ... )'
Word: '(', Confidence .179
Word: '...', Confidence .731
Word: ')', Confidence .885
Line: 'Networking'
Word: 'Networking', Confidence .995
Line: 'Identity'
Word: 'Identity', Confidence .959
Line: 'Location/Region r'
Word: 'Location/Region', Confidence .975
Word: 'r', Confidence .127
Line: 'L'
Word: 'L', Confidence .416
Line: '$ Cost analysis'
Word: '$', Confidence .63
Word: 'Cost', Confidence .993
Word: 'analysis', Confidence .994
Line: 'southeastasia'
Word: 'southeastasia', Confidence .993
Line: 'I!| Properties'
Word: 'I!|', Confidence .5
Word: 'Properties', Confidence .989
Line: 'Endpoint'
Word: 'Endpoint', Confidence .995
Line: 'Locks'
Word: 'Locks', Confidence .996
Line: 'https://southeastasia.api.cognitive.microsoft.com/'
Word: 'https://southeastasia.api.cognitive.microsoft.com/', Confidence .903
Line: 'V'
Word: 'V', Confidence .963
Line: 'Security'
Word: 'Security', Confidence .994
At CPI, we help many businesses develop AI solutions using Azure AI Services. Please contact us if you need assistance with your development.
Related Articles
Discover more from CPI Consulting Pty Ltd Experts in Cloud, AI and Cybersecurity
Subscribe to get the latest posts sent to your email.
Trackbacks/Pingbacks